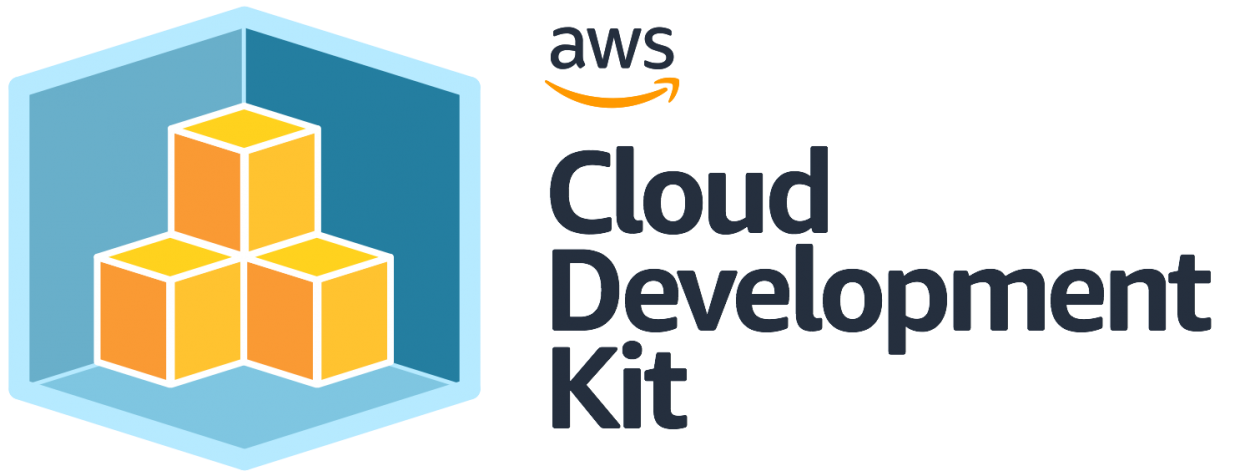
What is AWS CDK(Cloud Development Kit)? Learn with a example.
- Published on
- 4 mins read
What is AWS CDK anyway?
AWS CDK is a high-level object-oriented abstraction that simplifies the process of defining cloud infrastructure in AWS. It allows developers to use familiar programming languages to define cloud infrastructure as code, which can then be easily deployed to AWS. AWS CDK supports a wide range of AWS services and makes it easy to create and manage complex infrastructure.
Why use AWS CDK?
AWS CDK provides several benefits for developers:
Familiar programming languages: AWS CDK allows developers to use familiar programming languages like TypeScript, Python, and Java to define cloud infrastructure. This makes it easier for developers to learn and use AWS CDK.
Code reuse: Developers can reuse code across different projects and AWS accounts, reducing the amount of code they need to write and maintain.
Easy to maintain: AWS CDK automatically manages dependencies and keeps the infrastructure up-to-date, reducing the need for manual updates and maintenance.
Quick deployment: AWS CDK automates the deployment process, making it quick and easy to deploy infrastructure changes.
In this tutorial we'll be doing a TypeScript
demo to see it's application.
TypeScript: TypeScript is a popular programming language that provides static typing and object-oriented programming features on top of JavaScript. AWS CDK supports TypeScript, making it easy to define cloud infrastructure in a strongly-typed, object-oriented manner.
Let's take a look at an example of using AWS CDK with TypeScript to create a simple S3 bucket.
import * as cdk from 'aws-cdk-lib';
import * as s3 from 'aws-cdk-lib/aws-s3';
export class MyStack extends cdk.Stack {
constructor(scope: cdk.App, id: string, props?: cdk.StackProps) {
super(scope, id, props);
new s3.Bucket(this, 'MyBucket', {
versioned: true,
encryption: s3.BucketEncryption.KMS_MANAGED,
removalPolicy: cdk.RemovalPolicy.DESTROY
});
}
}
const app = new cdk.App();
new MyStack(app, 'MyStack');
This code defines an S3 bucket with versioning and
KMS
encryption. Thecdk.App
object represents the AWS CDK application, whilecdk.Stack
represents the stack that contains the infrastructure resources. Thes3.Bucket
object defines the S3 bucket and its properties.AWS CDK uses the construct concept to represent cloud infrastructure components. Each construct is a reusable building block that represents an AWS resource or a set of resources. In the example above, we create a new instance of the
s3.Bucket
construct, passing in thecdk.Stack
object as the first parameter, followed by the name of the bucket and its properties.The
removalPolicy
property specifies what should happen to the bucket when the stack is deleted. In this case, the DESTROY policy will remove the bucket and all its contents when the stack is deleted.
Deploying with AWS CDK
Once we have defined our infrastructure using AWS CDK, we can deploy it to AWS with just a few commands.
cdk bootstrap
cdk deploy
The cdk bootstrap
command sets up the AWS CDK environment in our AWS account, while cdk deploy
deploys the infrastructure defined in our AWS CDK code.
Thank you for taking the time to read my blog👍🏻. I strive to provide accurate and helpful information, but I understand that mistakes can happen. Please forgive any errors you may come across.
I value your feedback and would appreciate hearing from you if you notice any mistakes or have suggestions for future posts. Our goal is to continuously improve and provide the best possible content for our readers.
Thank you for your support and I look forward to hearing from you! 👍🏻🔥🦁🐻🐘🐬